When it comes to developer experience (DX) at ReadMe, two mantras often come to mind:
- Awesome by default 🆒 While developers often benefit from having a slew of configuration options and flags available in their tooling, too many options can feel overwhelming, particularly for first-time users. Having a sensible default experience will help your developers get to that first successful API call even faster.
- Meet users where they are 🤝 No two developers are alike, so your API (and your docs!) should reflect that. Your developers will often have different use cases, different technical stacks, and different levels of experience with APIs. The more your API can accommodate your developers and their diverse needs, the higher your API adoption.
With these in mind, we’re always looking for new ways to make APIs more accessible and lower the on-ramp to getting started. Today, we’re sharing more about api
, our open-source SDK generator.
api
takes your OpenAPI definition and generates a powerful SDK that’s custom-tailored to your API. With the world-class developer experience that an api
SDK provides, making API calls has never felt easier or more magical 🪄
The api
origin story 💭
You’ve probably heard a lot about APIs, but what exactly is api
and why does it matter?
Let’s start by looking at a typical code sample for an API call. In this example, the sample is written in JavaScript, and it uses the Fetch API to fetch our current job openings from the ReadMe API:
fetch('https://dash.readme.com/api/v1/apply', {
method: 'GET',
headers: { accept: 'application/json' },
})
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error('error:' + err));
ReadMe’s API reference automatically generates code samples for your endpoints in dozens of languages and libraries, including fetch
. And for good reason too — fetch
is popular, versatile, and it’s available everywhere!
- It’s baked into every modern web browser (including the one you’re likely reading this on!) 🌐
- It can run on the server out-of-the-box (thanks to runtimes like deno and Node.js 18) 📦
- It offers a tremendous amount of flexibility to handle a wide variety of API use cases 💪
But this approach isn't for everybody. Since fetch
and other generic HTTP clients are designed to make calls to nearly every API under the sun, there’s inherently going to be verbosity, boilerplate code, and a whole lot of configuration options. This makes your typical, off-the-shelf HTTP client pretty confusing for many API users.
At ReadMe, we’re constantly keeping an eye on the best DX out there, and we’ve come to notice a trend. Many of the top API-first companies out there offer their own custom SDKs, such as Stripe, Twilio, and Plaid (and I’m only linking to their JavaScript SDKs!).
Those companies and their respective SDKs are the tip of the iceberg. API-first companies are continually investing in SDKs for a variety of programming languages, and for good reason. With an SDK that’s custom-tailored to a single API, their users have much less boilerplate code and confusing configuration options to deal with, so they can get to that first successful API call even faster.
Thousands of great APIs run their developer hubs on ReadMe, where our customers are already documenting every little detail about their API using the OpenAPI Specification. This got us thinking: “the OpenAPI Specification already provides a ton of valuable information about an API, what if we use this to generate an SDK that’s as good as Notion’s JavaScript SDK”?
And that, my friends, is how api
came to fruition. 🌱
Making fetch api
happen 💄
Let’s revisit our previous example: fetching current job openings from the ReadMe API. The fetch
sample we looked at works perfectly fine, but what would an API call look like if the ReadMe API had a custom-tailored SDK? That’s where api
comes in.
Here’s what the same API call looks like with a code snippet using the api
-generated SDK:
readme.getOpenRoles()
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
Notice how there is far less boilerplate code than the fetch
example, and much of the complexity is abstracted away. But the code sample does the exact same thing!
Let’s break down the process of SDK generation, end to end:
- The ReadMe API is documented using an OpenAPI definition. Within that definition, there is an operation with an ID (i.e.,
operationId
) calledgetOpenRoles
. - Anytime an API user (in this case, someone that wishes to apply for a job at ReadMe) runs the installation command to set up the SDK,
api
takes the OpenAPI definition and generates an SDK that’s completely customized to the ReadMe API. It contains methods for every operation available in the API definition, as well as detailed descriptions of every request and response parameter. - Once the installation is complete, the user imports the SDK into their code and starts writing. Thanks to the comprehensive TypeScript definitions produced by
api
, code completion platforms like IntelliSense can guide them along as they write their snippet. They’re able to successfully hit the ReadMe API with a code snippet that’s a fraction of the length and complexity of the correspondingfetch
snippet.
That’s the developer experience ethos we’re going for with api
: a readable, succinct code snippet that will get you to that first successful call in a jiffy. 🥜
Elevate your OpenAPI-powered DX with api
📈
One important design consideration as we built api
is that we wanted the SDK generation to be completely agnostic of your documentation platform, much like OpenAPI itself. Because api
is built on top of the OpenAPI specification, anybody that describes their API using OpenAPI can step up their DX with a custom SDK generated by api
.
There are a few requirements to get started:
- Node.js and
npm
installed - A local directory with a
package.json
file created - An OpenAPI definition, either located in your directory or served from a URL
Once you have everything in order, you can generate a custom-tailored SDK by running the following command from the root of your directory and following the prompts:
npx api install [path-to-api-definition]
Once the SDK is generated, you’ll be writing succinct code snippets and getting to that successful first API call in no time 🚀
Two birds 🐦, one incredible DX 🌟
If there’s one thing that developers, technical writers, and pretty much anyone enjoys, it’s being able to stretch your work so it goes further and saves you time. And that’s another reason why we’re so excited about api
and how it leverages the existing OpenAPI ecosystem to supercharge your users’ developer experience in ReadMe.
Before api
came around, there were already many great ways that a comprehensive OpenAPI definition could set your API reference apart and further set your users up for success:
- Comprehensive security scheme definitions can help your users navigate one of the trickiest parts of getting started with an API: authentication!
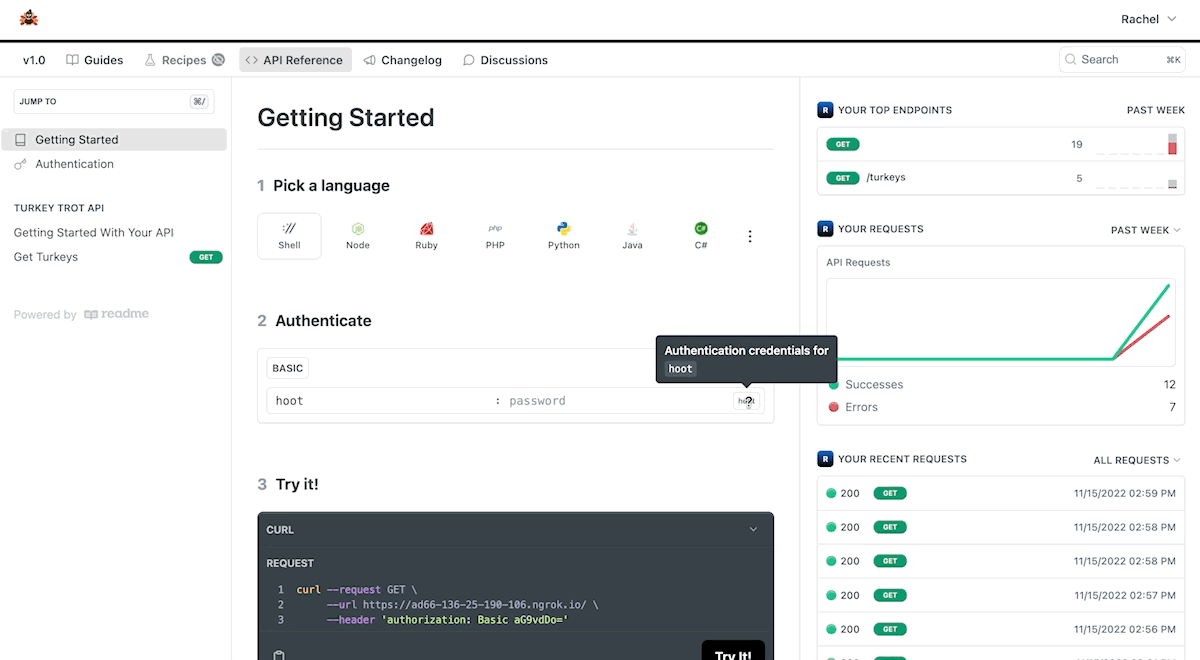
- Detailed request parameter descriptions and definitions (with default values, enums, and examples!) eliminate ambiguity and help your users understand exactly what data to send to your API:
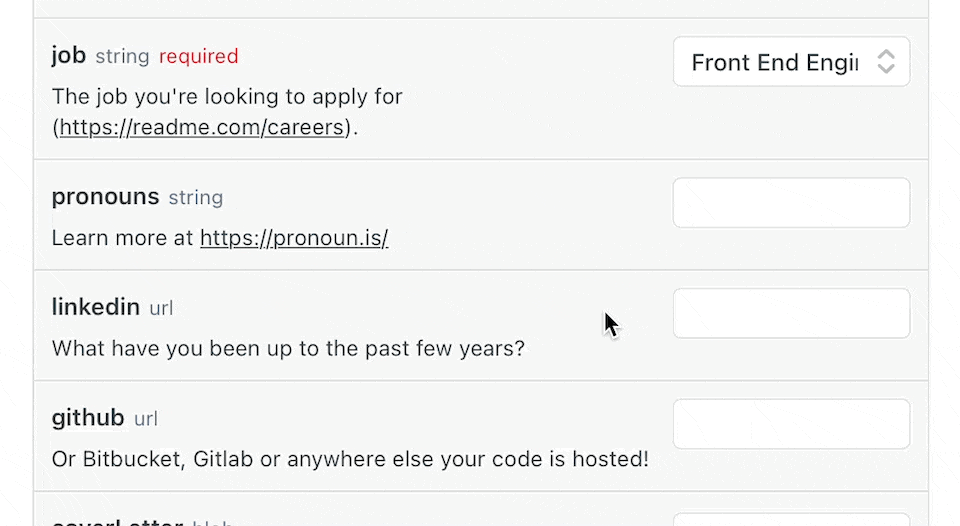
- By capturing exhaustive response schemas and plenty of examples for every edge case, your users will know exactly what to expect from your API’s responses:
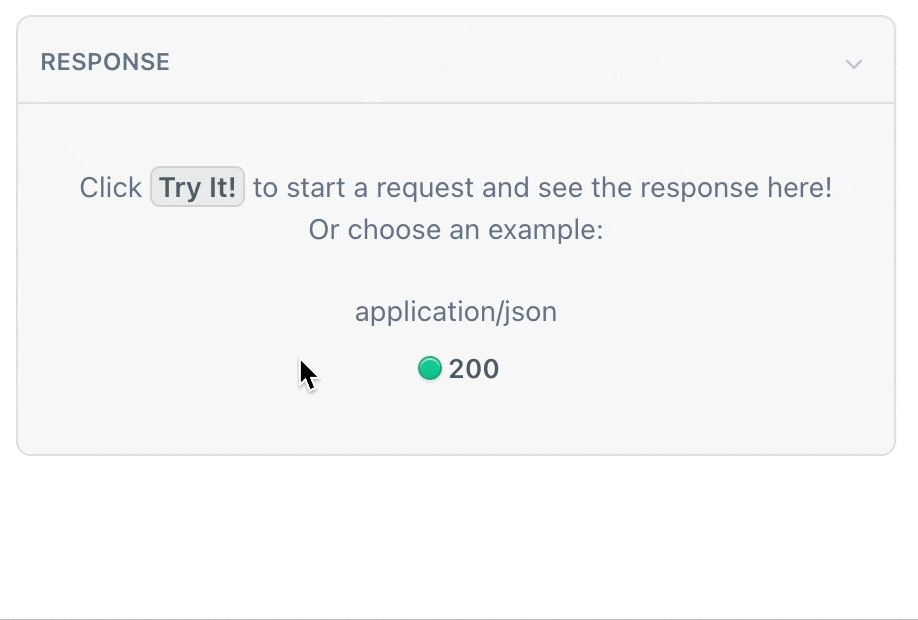
But who said that a great developer experience should start and end with your docs? By investing in the above aspects of your OpenAPI definition, your users’ experience with your api
-generated SDK becomes even more magical:
- Thanks to that security scheme definition, passing in authentication credentials into your SDK is a one-liner:
sdk.auth('API_KEY');
- Because of those detailed request parameter definitions, passing in request parameters is a much more straightforward exercise that uses a fraction of the boilerplate code. And thanks to the power of TypeScript, users will get helpful cues about the parameters as they write in their editor:
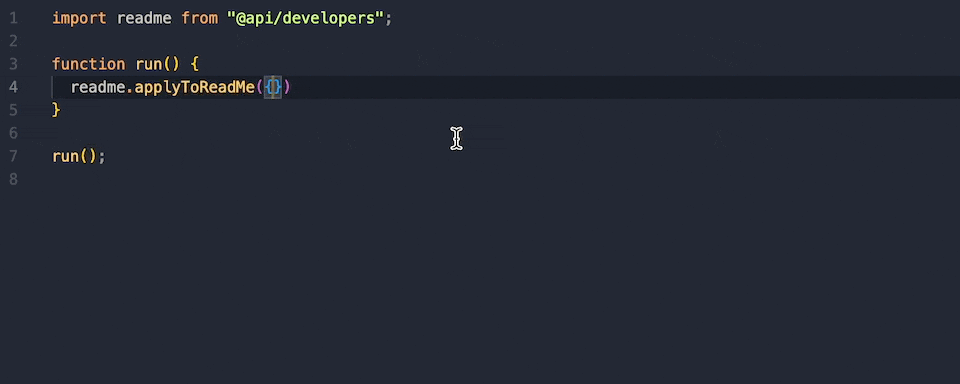
- And because of those exhaustive response schemas, users will also be able to leverage TypeScript hints to parse out response bodies:
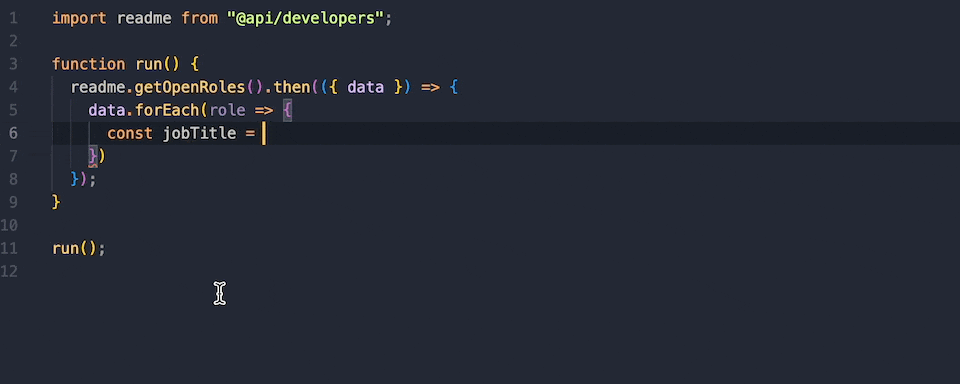
There’s already a tremendous amount of value in putting together a rock-solid OpenAPI definition. With api
, you get even more developer experience bang for your OpenAPI buck 💸
Available now in your ReadMe API reference 🦉
If you have an API reference section that’s powered by ReadMe, your users can start writing better API calls with api
today. They can select Node in the language selector and select the api library to get started.
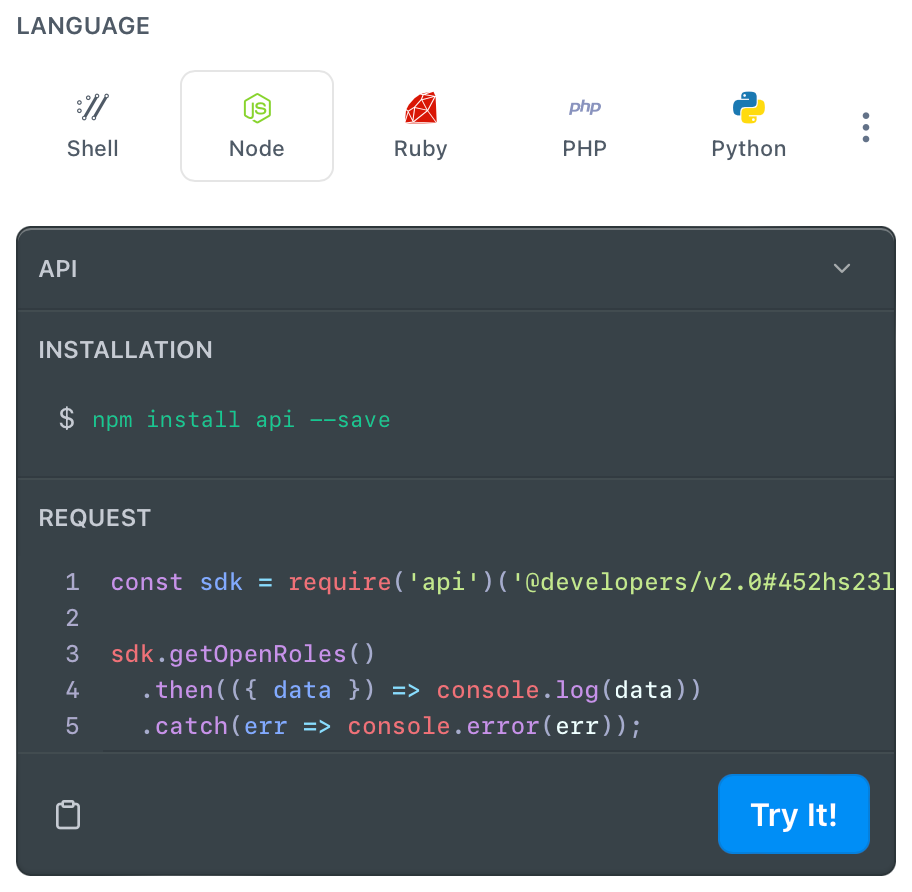
If you run into any issues, check out our docs and feel free to contact us at support@readme.io. api
is proudly open-source (shoutout to Jon for your all your hard work on this!) so feel free to open up an issue in the api
GitHub repository.